Below are few useful git commands that can help and ease our git experiences.
Git Initialization
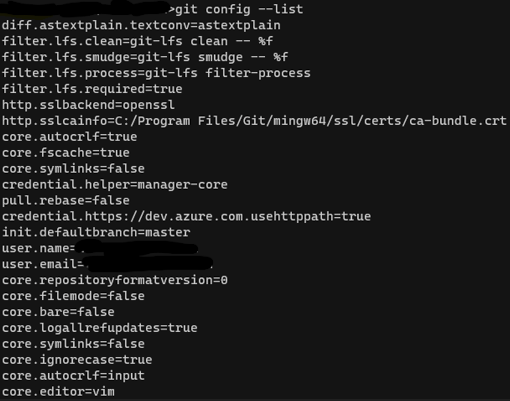
All files from the repo (which should have LF line endings in this scenario) are converted to CRLF line endings on checkout to a Windows PC. All files are converted back to LF line endings on commit from a Windows PC.
There can be issues if we have binary files. Then better option will be to set .gitattributes for specific files
Git Remote
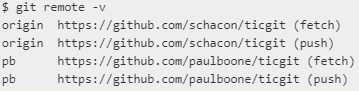
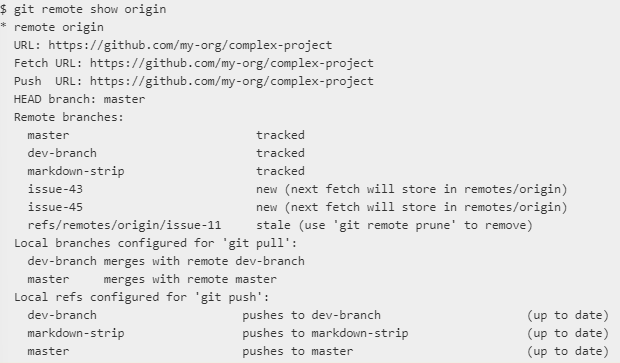
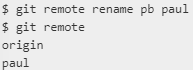
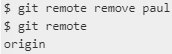
Git Branch

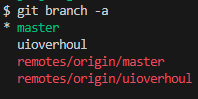

Switches back to previously used branch.
Merge feature-branch to the current branch and creates a merged-branch.
We have to fire git commit -m "feature and master merged" to commit merged changes
Brings latest change in master to current branch
Current branch now bases on the latest changes in master branch
We can use below in short as well.
git push -u origin new-branch-name
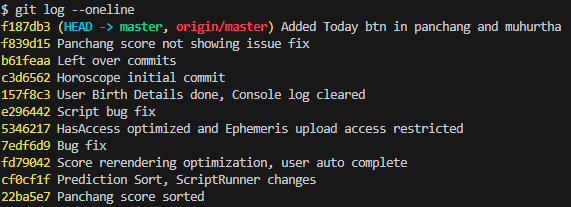
Get user, time of commit, and other details in log output.
0fc8fca Author name Sat Sep 30 07:33:04 2023 +0530 Commit Message
%h = hash (commit hash)
%an = author name
%ad = author date
%d = ref names
%s = subject
-5 = show only last 5 lines
Get user, time of commit, and other details with colour in log output.
6c3e1a2 Sat Sep 30 07:33:04 2023 +0530 Author name lsof is a dependency now.
%h = hash (commit hash)
%cd = committer date (format respects --date= option)
%aN = author name (respects .mailmap)
%d = ref names
%s = subject
-5 = show only last 5 lines
Provided we have not pushed the code to remote yet.
git commit --amend --no-edit
Provided we have not pushed the code to remote yet.
Provided we have not pushed the code to remote yet.
Provided we have not pushed the code to remote yet.
Git Work In Progress

Brings latest changes in master into current branch.
Current branch now bases on the latest changes in master branch.